Introduction
This page is just an assortment of tiny experiments - mostly how I learned (and still do) shaders. I started (and spent a long while) in Shadertoy which is a wonderful community of people sharing demo shaders. Some of them are absolute magicians and make astonishing stuff!
The creator of shader, Inigo Quilez also has many useful articles and tutorials on his website, so do check him out! Someone else to keep in mind is Alan Zucconi who also has some useful tutorials, especially when dealing with Unity as well.
As I said, these are tiny experiments, and mostly proofs-of-concept. At the very least, they let me get my hands dirty and take the first step into implementing some effects I saw in games.
Note: most of these examples are static pictures that link to a dynamic shadertoy shader. Click on them to see the full effect!
Update: I've had to opportunity to apply what I've learned in these experiments in The Magic Flute game! Check it out if you want to see some of these effects used in the context of an actual game.
Noise
As the title indicates, these shaders are mostly about noise. I still find it really cool the number of creative uses for noise in texture synthesis, and these shaders are my own forays into it. They use various kinds of noise, like Perlin Noise, Fractional Brownian Motion, Ridged Noise, Domain Warping, Voronoise and sometimes different combinations depending on the effect.
3D Marble
This idea popped into my mind as I was walking through Anor Londo. The cool thing, to me, is that using 3D noise, you can make 3D textures - which means you can cut up a block in any way you want! You could make a whole Greek Temple with a single marble block, with every single texel matching. It can also be used to deform 2D textures (in animations) or to create seamless texture (by mapping the texture onto a torus/donut).
Now that I look at it years later, it can be polished up quite a bit, but I liked the idea anyway. I used two noise textures, slightly offset (on the third dimension) and averaged, to create more "smudged" black cracks. It was an improvement over the earliest marble textures from textbooks, at least, but there is obviously much better work nowadays.
Ridged Lava
Simple lava using a similar idea to the Marble's - two layers of ridged noise, slightly offset and averaged. Would probably benefit from some swirling noise like domain warping.
Ridged Warping
I must confess I didn't even remember writing this shader, but I like it! Looking at the code, it is simple domain warping with ridged noise instead of fbm.
Domain Warp Distortion
This is straightforward domain warping applied to a texture, but changing its coordinates rather than color. It is mostly a proof-of-concept kind of thing, but I am a sucker for distortion effects in general, especially on explosions and sword slashes and so on. This is also the kind of distortion used in The Witcher 2, during the eternal battle.
Eyeris
Domain warping with a radial color palette, looks good in motion. The idea came from the game Back to Bed, while I was proving its PSPACE-Completeness for my thesis.
I actually went back to this one in The Magic Flute, check the bottom of that page to see the result.
Planets
These are from when I was working on the Nightsky project. Straightforward application of noise, sometimes with vertex displacement as well. Looks particularly good on gas giants (although some fluid dynamics also work great).
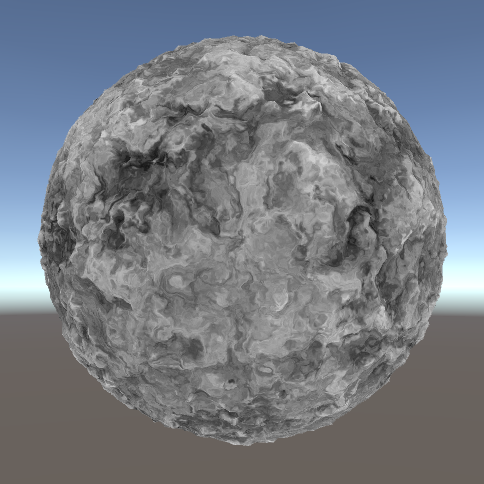
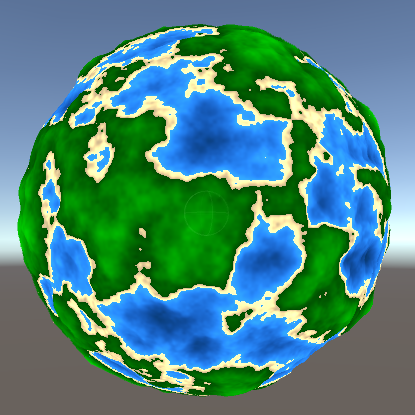
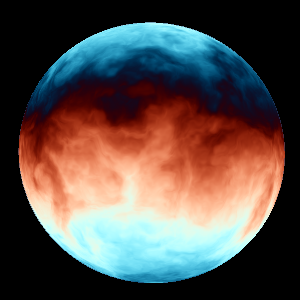
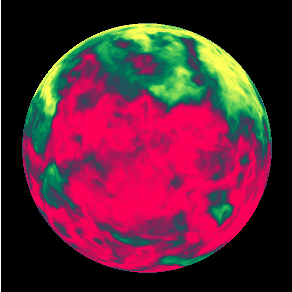
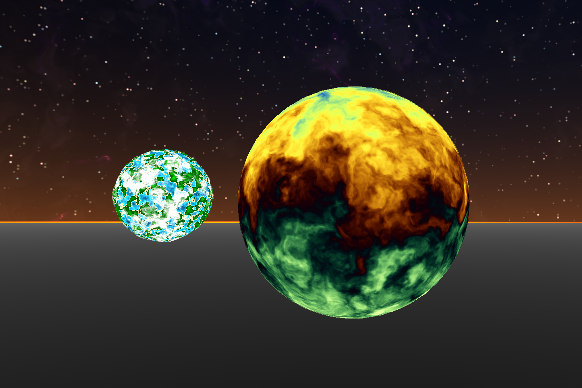
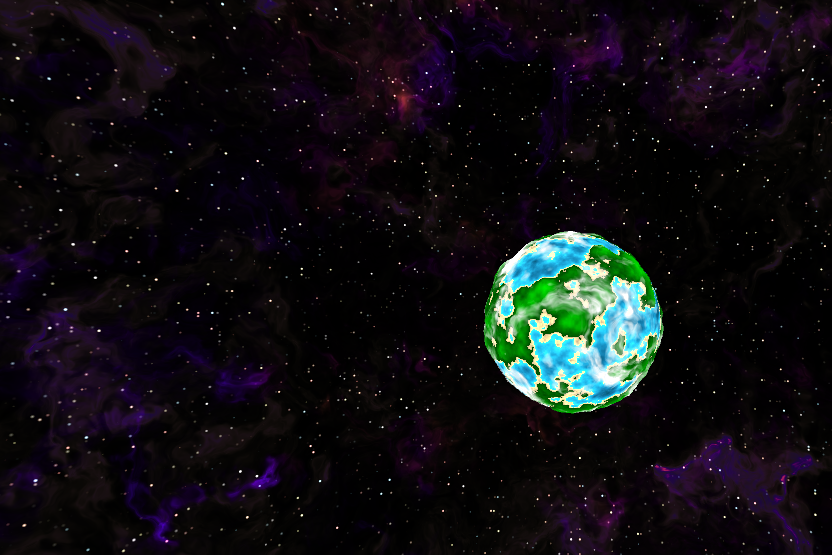
Ray Marching
Ray Marching is like the shader equivalent of Ray Tracing. You essentially send a ray for each pixel of the camera and render the result. I have mostly used it to render implicit surfaces, like distance fields or heightmaps. I particularly like the way you can smoothly blend different shapes using it, unlike the traditional mesh approach. There are also some astonishing examples of 3D fractals as well, which I'd love to look into at some point (for uses in procedural architecture, for example) but have yet to do so. Volumetric clouds are another area I'd like to look into, eventually, but there are always more ideas than time (and motivation) to implement them!
Iridescent Blob
This is fairly simple - it's just a sphere, with a couple of modifications. The first is the use of noise to modify its surface and make it blob-y. The second is to change its hue depending on the light and viewing angle - a kind of fake iridescence.
Tower of Timbuktu
As I was just learning ray-marching, my father mentioned Timbuktu's architecture to me (for some reason). When I saw it, it immediately reminded me of the smooth shapes possible with distance fields, so I made a small tower as an example. It's a simple trapezoidal prism, blended with a sphere at the top, and negatively blended with a box for a door. FBM noise is then applied to the surface to make it look sandy.
Fuji
Well, not really Fuji. Just a snowy volcano. It was a combination of Ridged Noise, a truncated cone in the middle for a height, and then an erosion algorithm, the same I mentioned in the erosion project. The snow is just a white color when the surface normal is upward enough.
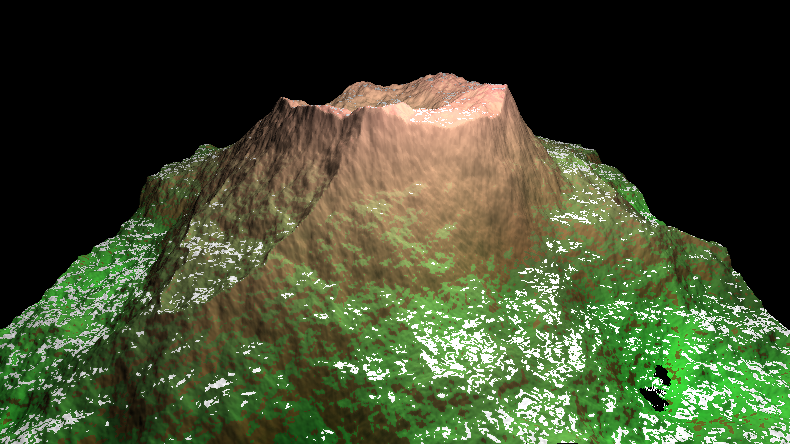
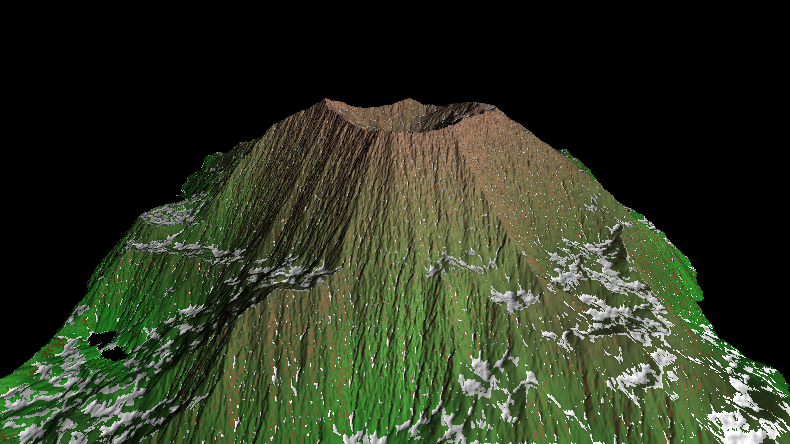
Buffers
This section is dedicated to more complicated shaders, namely ones that are not applied directly to a texture or the screen, but use auxiliary buffers (like depth and normal buffers, and so on). It is typically used for post-processing rendering, like blur, cel-shading, outlines, and so on. It is still rather empty, since I haven't had the opportunity to experiment much with it, but I'll probably get to it at some point!Sun-shafts/God rays
I think this is a pretty common trick to render god rays. First, you render the whole scene into a silhouette (render every opaque object black, and semi-transparent objects shades of gray), with light sources in lighter colors. Then, you apply radial blur to the dark areas, away from the light, and it gives the effect of soft shadows.
This scene was deliberately low-res, since it was part of a different project that I was working on, also in low res. The clouds are simple FBM noise and are animated.
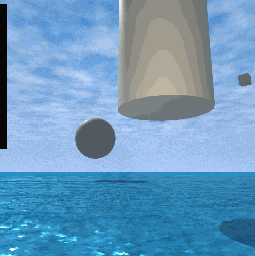
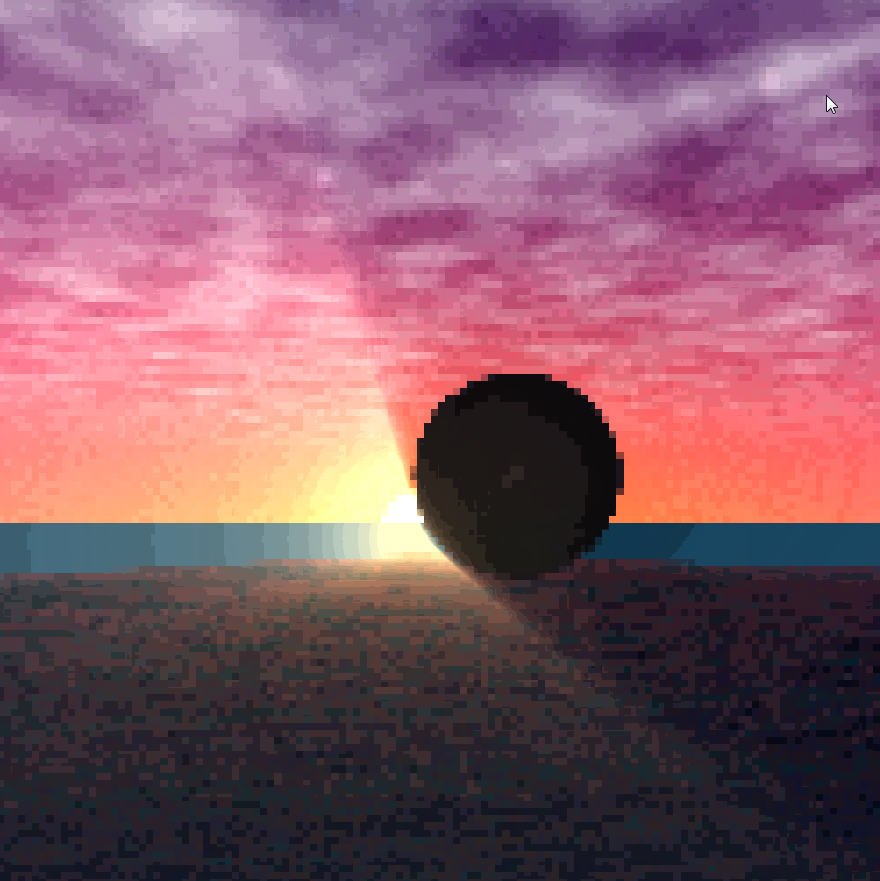
This was an offshoot of the Angkhorror game.
Others
Reaction-Diffusion
Reaction-diffusion systems hold a special place in my heart, and I hope to go back to them someday. The basic idea is to simulate the reaction of 2 different chemical on a surface. The fascinating thing is that it is a kind of morphogenesis, the subject of one of Alan Turing's last papers.
They are also similar to cellular automata, and exhibit the kind of pattern seen all of nature, especially in fish and coral, for example. I'd love to use these in procedural generation some day - with today's GPUs, they are ripe to be used for earthly and alien creatures alike! Check, for example, Greg Turk's brilliant PhD thesis when applied to meshes, or these two books, from the same collection as the wonderful Algorithmic Beauty of Plants on L-Systems.
Below are just few superficial examples, with varying parameters. The first three you can interact with on shader toy - and I find it extremely satisfying to click and drag the mouse and see the patterns readjusting! The last two I made in Unity, so no interaction for you.
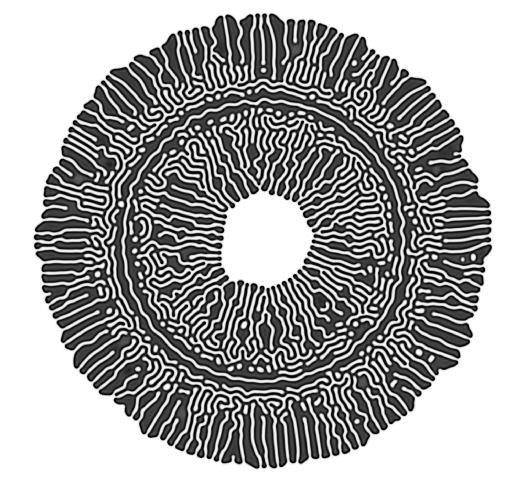
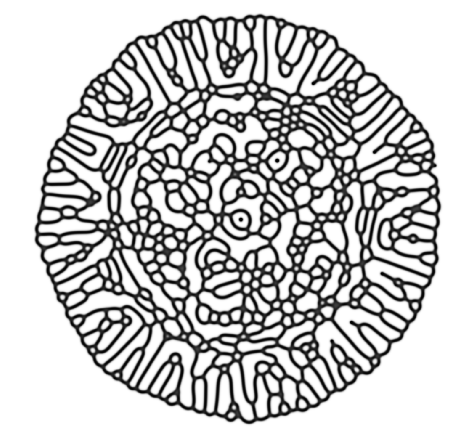
Simple Mandelbrot
Fractals are the bread and butter of computer graphics, but I had never tried to render any. I was reading Benoit Mandelbrot's autobiography and got the gist of the computation, so I tried to implement it in a shader. It's nothing special and has some artifacts, but it captures the main idea.